PHP - The Stuff you need
to Know
PHP is one of the easiest server side languages to use on the
web. Just because it's easy, doesn't mean that it isn't powerful
and fast. The great thing about PHP is it is a non compiled
language like html or javascript so if you already know html
or JavaScript, it's the next natural progression for you. There
are very few things that you can't do in php than you can do
in a compiled language as far as the web is concerned. I think
once get a few basic scripts under your belt, you will see how
useful and easy php really is.
This lesson is not comprehensive in php. If you want to learn
more about php go to http://www.php.net/
or buy a good book. Here are a few basic scripts to get you
going. As you learn PHP, you will find that there other and
often better ways to do what we are doing here. Here we will
keep everything to a minimum and try to make the examples useful
for your web sites so you can build on a firm foundation (you
understand) as well a make use of these scripts immediately
on you current projects.
Hello World
The first script is pretty useless but it does show you how
easy it is to write code in php. Here is the classic example
"hello world."
Open Dreamweaver.
File > New
In the General Tab area, select Dynamic Pages and then php.
Click create.
In the insert bar select php.
Select Code View
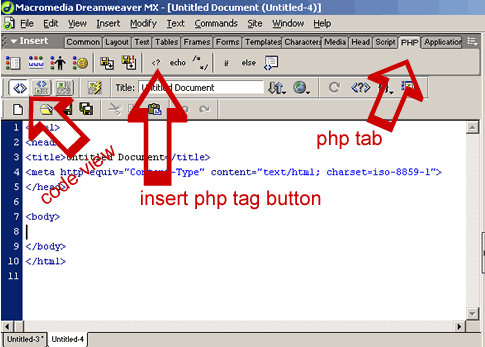
Save your file as helloworld1.php
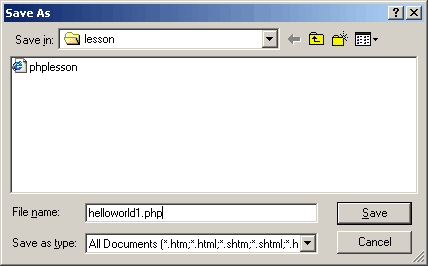
Click on the little button that says echo in the php insert
bar.
Then type in the parentheses and the words "hello world."
<?php echo "hello world" ?>
If you save your file again and upload it to the Internet,
you will see hello world printed on your page. Pretty easy,
right?
Note: Unless you have php installed on your local computer,
you will not be able to see your work until you upload it to
your Internet server. PHP must be installed on your Internet
Server by the server administrator.
Now lets make it a little better.
Click on the echo button again and type "<br>the
time and date are<br>"
<?php echo "<br>the time and date are<br>"
?>
then click in the echo button one more time and then type in
date("H:i,jS F");
<?php echo date("H:i,jS F"); ?>
Your complete script should now look like:
<?php echo "hello world" ?>
<?php echo "<br>the time and date are<br>"
?>
<?php echo date("H:i,jS F"); ?>
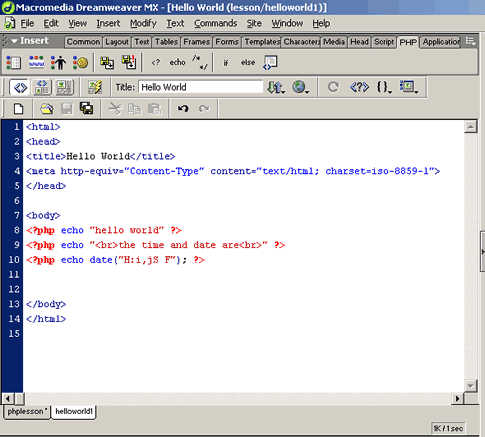
Upload it to your server and view in on the Internet.
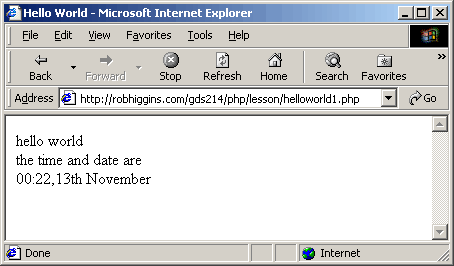
What did we just do here?
First we used the echo command to display our text "hello
world".
<?php echo "hello world" ?>
This statement contains two parts:
All php statements start with php tags. There are several php
tag styles:
- Short Style - example: <? echo "hello world";
?>
- XML Style - example: <?php echo "hello world";
?>
- Script Style - example: <SCRIPT LANGUAGE='php'> echo
"hello world"; </SCRIPT>
- ASP Style - example: <% echo "hello world";
%>
Dreamweaver uses the XML style as a default so that's why we
used it. It's easy.
The second part, the echo command, is one way to print to the
web page. Anything that you put between the parentheses will
print on the page. Notice that you can put html code between
the parentheses. Notice that we inserted out php code in the
body of the document in a regular html page. This is what is
so cool about php. It is almost like an extension of html.
The date() function is a built in function of php. The H is
the hour in the 24 hour format, i is the minutes with a leading
zero where required, the coma is a coma, the j is the day od
the month, the s adds the ordinal suffex (in this case the "th")
and the F is the year in four digit format. (for more information
about the date() function go to the web or a php reference book).
<?php echo date("H:i, jS F"); ?>
Notice that we pass a string (inside parentheses) to a function
date(). This is called the functions parameter or argument.
Now with that out of the way, we'll get started on something
useful, a html form that updates the content on a web page.
Updatable web pages
Typically, my customers like to have one or more pages that
they can update themselves with little on no html experience.
An updatable web page is just that. It's a form that only the
customer has access to, a php file, a text file that stores
the data, and the html page that displays the information to
the world.
It sounds complicated but in reality it is very easy. The amount
of code in each file is minimal and you can put up an updatable
page in very little time.
What we are doing here is installing a php script right in
the part of your html page that you want to update. I typically
do it in a table cell. The choice is yours. For this exercise
we won't get very fancy but you will be able to use this code
in an html page layout that you wish to add and update content
to through a form.
The form can also be an exact replica of the web page except
where the updatable text is there is a form field but it doesn't
have to be. It can be just a simple form. Depending on how much
the customer is spending is how I draw the line. If there not
spending much, I'll just do a siple form.
I guess the first place to start is with the form. All we need
is a simple form with one text area for this exercise. In real
life, you may wish to use more than one updatable field. If
you do, these same principle apply. Just add more fields to
your form, your script and the resulting html page.
Here is the form. I just inserted a form in Dreamweaver, added
one text area box called content, added a submit button and
then called my update.php file (which we will make in a minute.



Here is the code in code view:
<form action="update.php" method="post"
name="update" id="update">
<p>
<textarea name="content" cols="60" rows="10"
id="content"></textarea>
</p>
<p>
<input type="submit" name="Submit" value="Submit">
</p>
</form>
Save your form and call it update.html
Note: In real life, you would most likely insert this form
inside a copy of the web page you would want to update. Just
delete the stuff you want to replace and then paste in the form.
You page should look just like it always did except the form
field will replace the content you want to update.
The php page
Now we will write our php code that will write the content
that we submit from our form to a text file on our server. The
html page that the world sees (we'll be making that in a minute)
will read from the text file this program creates.
Here is the code:
<?php
$outputstring = $content;
$fp = fopen("text/update.txt", "w");
fwrite($fp, $outputstring);
fclose($fp);
echo $content. "<br>";
echo "<p><a href='updated.php'>see it on the
web?</a></p>";
?>
Here is what we did in our little script:
The first thing of course is the php tag.
Next we put our content field (which is recieved as $content
by php) into $outputstring
Next we open a file for writing in the folder text called update.txt
and read the contents into $fp
Then we write $outputstring which is now inside $fp to the
text file which replaces any previous content in the text file
update.txt (we used the w when we opened the file which means
overwrite).
Then we echoed the $content to the page so we could see what
we just wrote for proofreading.
And finally we echoed an html link to the actual page we will
use to see it on the web (which we will create next).
The Viewing Page
Create a new php page or open the html page that you want to
update and save it as .php in Dreamweaver as updated.php.
Whereever you want to place your updatable text, place your
cursor in code view.
Insert the following php code:
<?php
$fp = fopen("text/update.txt", "r");
while (!feof($fp))
{
$content= fgets($fp, 100);
echo $content."<br>";
}
?>
Here's what we did...
we opened a file pointer called $fp for reading only (thus
the "r") for the file update.txt in our folder text.
while (!feof($fp)) is a while loop that says as long as the
file pointer $fp is not at the end of the file (feof is easier
to remember if you know it means file end of file), keep going
until it is.
Then we used the fgets to get the content in $fp into $content.
The 1000 is the limit of characters than can be read.
And finally we echo the $content to the web page.
Save your page and upload it to the web.
It's very basic but that's what you wanted, right? It's up
top you to create the html code but just add the script and
you are in business.
Here is alll the code:
update.zip
|